Object List
Often, you need to group objects with a common property or type, or with a common goal in the game. For example, you need to group the enemy objects, the pickable items, the platform objects, so you can test if the player collides with them.
For this purpose, the Scene Editor allows grouping the objects of the scene into a JavaScript array.
You can create an Object List by dropping a List built-in block on the scene:
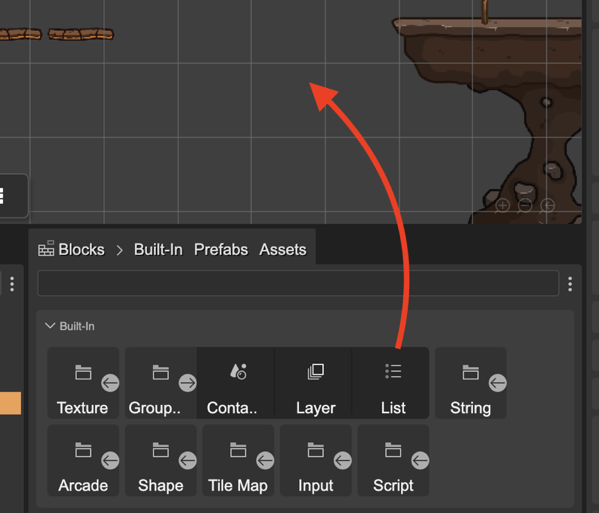
Or you can create a new list when adding an object to the list:
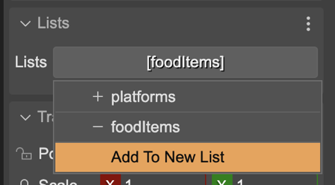
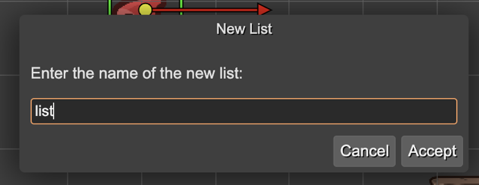
To add an object to an Object List, select the object and look for the Lists section. Click on the drop-down of the Lists property, it shows the lists availables in the scene with the option of add the selected object to a list or remove it from a list:
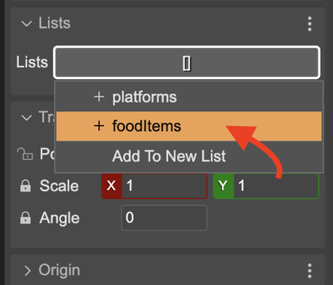
The Outline view shows the Object Lists of your scene, in the Lists category. It also shows the objects of every list:
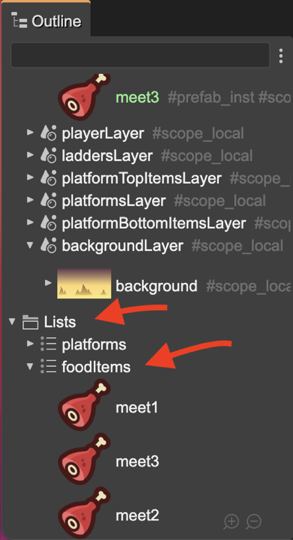
You can remove items from a list by selecting them in the outline and pressing the Delete command (Del
).
Also, you can sort the items in the list with the Move commands, just like you order the game objects in the scene:
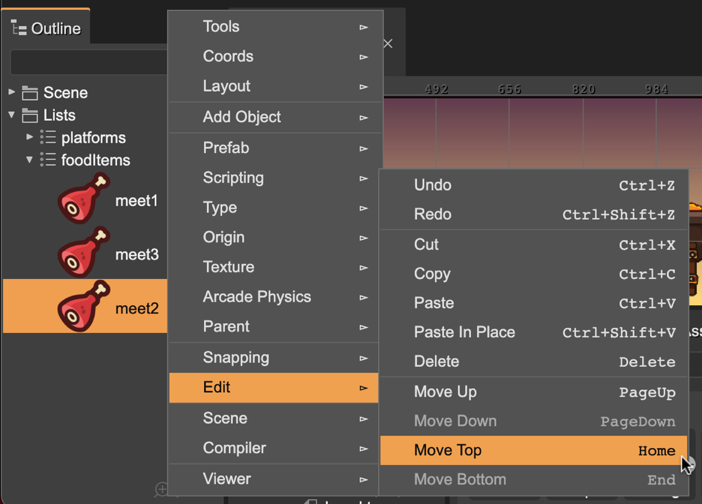
You can locate the a list item in the scene. Select the list item, and in the List Item section of the Inspector view, click on the Select Game Object button:
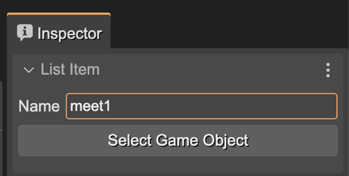
Object List properties
The Object List type is not part of the Phaser API, it is something introduced by the Scene Editor. In the Variable section of the Inspector view, it shows the Variable properties:
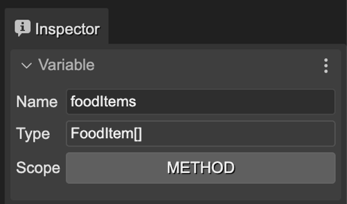
In this section you can modify the name of the list, see the inferred type of the list items, and change the scope (CLASS
by default) of the object list variable.
Object List code generation
The code generated by the scene compiler, to create a list, is like this:
this.pickableItems = [melon, melon_1, melon_2, bomb, bomb_1, bomb_2];
If the output language is TypeScript, the scene compiler generates a field declaration for the list:
private pickableItems: Array<Melon|Bomb>;
Note that the scene compiler infers the type of the array elements: a union of Melon
and Bomb
, that are prefabs. It also infers the type of the elements if are Phaser built-in types:
private parallax: Phaser.GameObjects.Image[];
This detailed type declaration of the arrays allows that code editors like Visual Studio Code can provide a smarter coding experience.
Object List vs Phaser Group
Traditionally, Phaser uses the Group game object to join objects around a common purpose. But grouping is not the only feature of a Group game object:
It implements an object pool.
Is a data structure with methods for sorting and iterate the items.
Can be used as a proxy to modify the state of the items.
To call a method of the items.
To apply game-related global operations to the items.
So, why the Scene Editor is not using the Group game object?
Many of the features of a Group game object are especially helpful to implement the logic of the game, but what a level maker needs is just to organize the objects, we think.
The Group game object type is not parameterized. A code editor type-inference/auto-completion engine works much better with a simple JavaScript array. When you write
const enemies = [enemy1, enemy2, ...]
, a code editor like Visual Studio Code can infer the type of the array items.Even for a human, a simple JavaScript array could be more comprehensible.
If you need a Group game object, you can create it with the array of objects generated by the Scene Editor:
const pickableItemsGroup = this.add.group(this.pickableItems);